Breadcrumps
Renders a breadcrumb component that displays the current page's path. This is a React component written in Typescript, styled with Tailwind CSS that can be copy & pasted into your codebase.
Step-By-Step Guide
1. Install Dependencies
Icons by Lucide. But you can use any or none.
cli
1npm install lucide-react
Copy the function 'cn'. Link -> cn Function
Copy the function 'capitlize'. Link -> cn Function
2. Copy the Source Code
@/components/breadcrumps.tsx
1'use client';
2
3// Import Types
4// Import External Packages
5import { usePathname } from 'next/navigation';
6import Link from 'next/link';
7// Import Components
8// Import Functions & Actions & Hooks & State
9import { capitalize, cn } from '@/lib/utils';
10// Import Data
11// Import Assets & Icons
12import { ChevronRight, HomeIcon } from 'lucide-react';
13
14/**
15 * Renders a breadcrumb component that displays the current page's path.
16 * @returns The breadcrumb component.
17 */
18export default function Breadcrumps() {
19 const pathname = usePathname();
20 const pathArray = pathname.split('/');
21
22 return (
23 <div className="flex items-center py-4 text-sm text-muted-foreground">
24 <Link href="/" className="px-2 sm:px-0" aria-describedby="home button">
25 <HomeIcon className="h-5 w-5 sm:h-4 sm:w-4" />
26 </Link>
27
28 <div className="flex flex-wrap">
29 {pathArray.length > 1
30 ? pathArray.slice(1).map((path, index) => (
31 <div key={path} className="flex items-center leading-10">
32 <ChevronRight className="h-4 w-4" />
33 <div
34 className={cn(
35 pathArray.length - 2 === index
36 ? 'font-medium text-foreground'
37 : 'text-sm text-muted-foreground'
38 )}
39 >
40 <Link href={pathArray.slice(0, index + 2).join('/')}>
41 {path
42 .split('-')
43 .map((w) => capitalize(w))
44 .join(' ')}
45 </Link>
46 </div>
47 </div>
48 ))
49 : null}
50 </div>
51 </div>
52 );
53}
3. Use in your App
page.tsx
1import Breadcrumps from '@/components/Breadcrumps';
2
3export default function Page(){
4 return(
5 <Breadcrumps/>
6 )
7}
Tech Stack
Only the finest ingredients are used in our products. We made sure that we only use the best technologies available which offer a free tier! Yes, you read that right. Everything can be set up for FREE!
Example Usage
See this component live in action. Are you using the component on your website? Shoot us an email (support@boilerplatehq.com) and we will link to you.
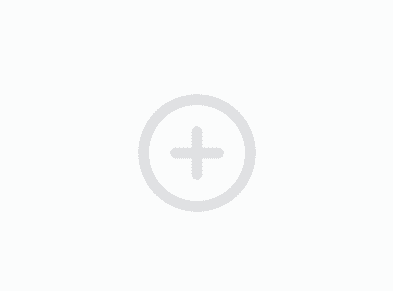
Your Website?
Are you using this component? Shoot us an email and we will link to you.
You May Also Like
Didn't find what you were looking for? Check out these other items.
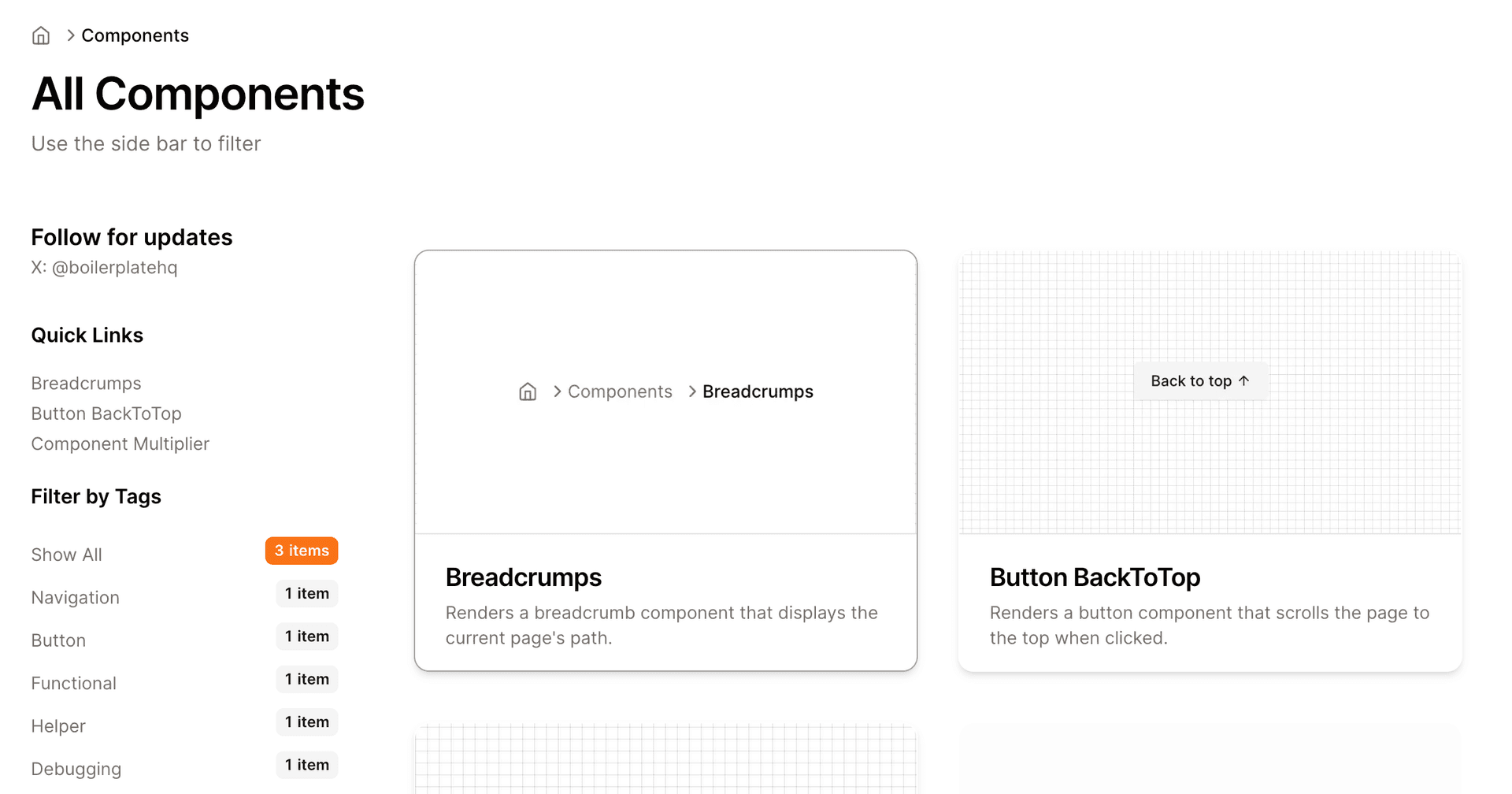
Ui Component Library
Want to create your own Ui Component Library like shadcn/ui? This is your template!
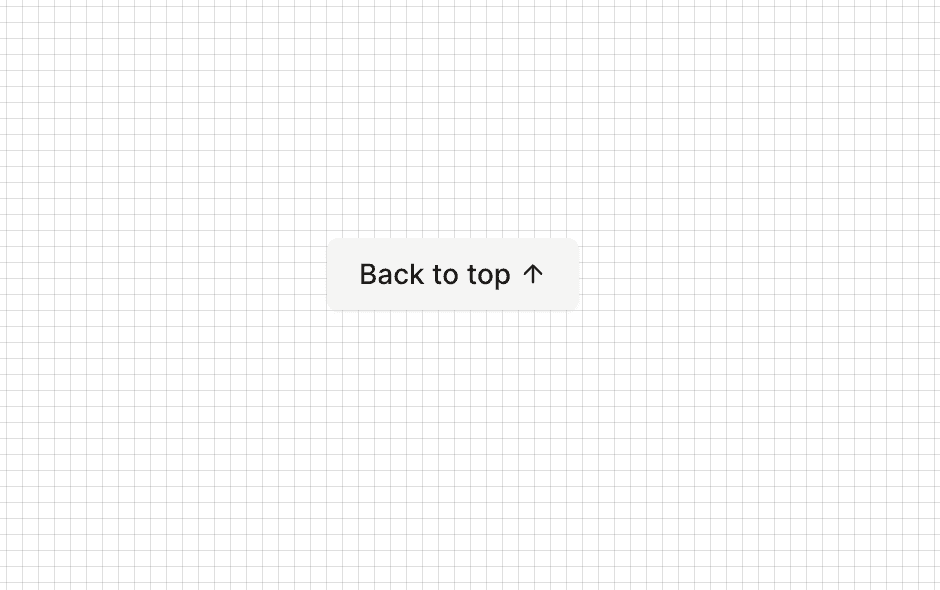
Button BackToTop
Renders a button component that scrolls the page to the top when clicked.
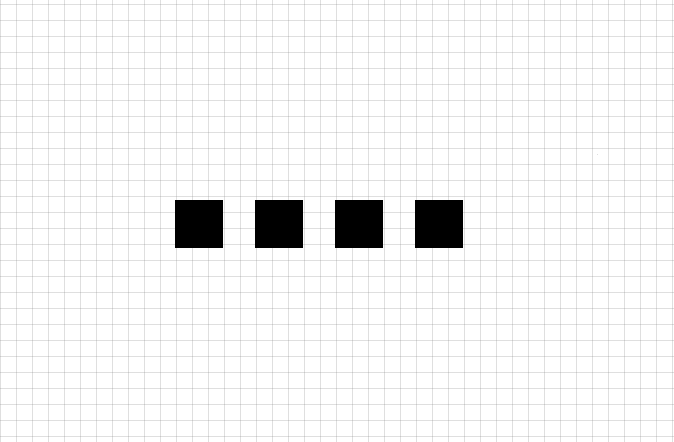
Component Multiplier
Renders multiple instances of a given component, e.g. for testing purposes.
Frequently Asked Questions
We have compiled a list of frequently asked questions. If you have any other questions, please do not hesitate to contact us via email or using the chat function. We are here to help!