cn
Combines multiple Tailwind class names into a single string. This is a utility Typescript function that can be copy & pasted into your codebase.
Step-By-Step Guide
1. Install Dependencies
cli
1npm install clsx, tailwind-merge
2. Copy the Source Code
@/lib/utils.ts
1import { type ClassValue, clsx } from 'clsx';
2import { twMerge } from 'tailwind-merge';
3
4/**
5 * The e=mc^2 of Tailwind. Combines multiple class names into a single string.
6 *
7 * @param inputs - The class names to be combined.
8 * @returns The combined class names as a string.
9 */
10export function cn(...inputs: ClassValue[]) {
11 return twMerge(clsx(inputs));
12}
3. Use it in a file
/page.tsx
1import { cn } from '@/lib/utils';
2
3export default function SomeComponent(){
4 return (
5 <div className={cn('w-48 bg-rose-400', true&&'h-48')}></div>
6 )
7}
3. Examples
/page.tsx
1// Pass down classname props from a high level component
2import { cn } from '@/lib/utils';
3
4export default function SomeComponent({className}:{className?:string}){
5 return (
6 <div className={cn('py-2', className)}></div>
7 )
8}
9
10// Toggle classes conditionally
11'use client';
12import { useState } from 'react';
13import { cn } from '@/lib/utils';
14
15export default function SomeComponent() {
16 const [bgIsRose, setBgIsRose] = useState(true);
17
18 return (
19 <button
20 className={cn('py-2 w-48 h-48', bgIsRose && 'bg-rose-500')}
21 onClick={() => setBgIsRose(!bgIsRose)}
22 >
23 {bgIsRose ? 'Remove Rose' : 'Add Rose'}
24 </button>
25 );
26}
Tech Stack
Only the finest ingredients are used in our products. We made sure that we only use the best technologies available which offer a free tier! Yes, you read that right. Everything can be set up for FREE!
You May Also Like
Didn't find what you were looking for? Check out these other items.
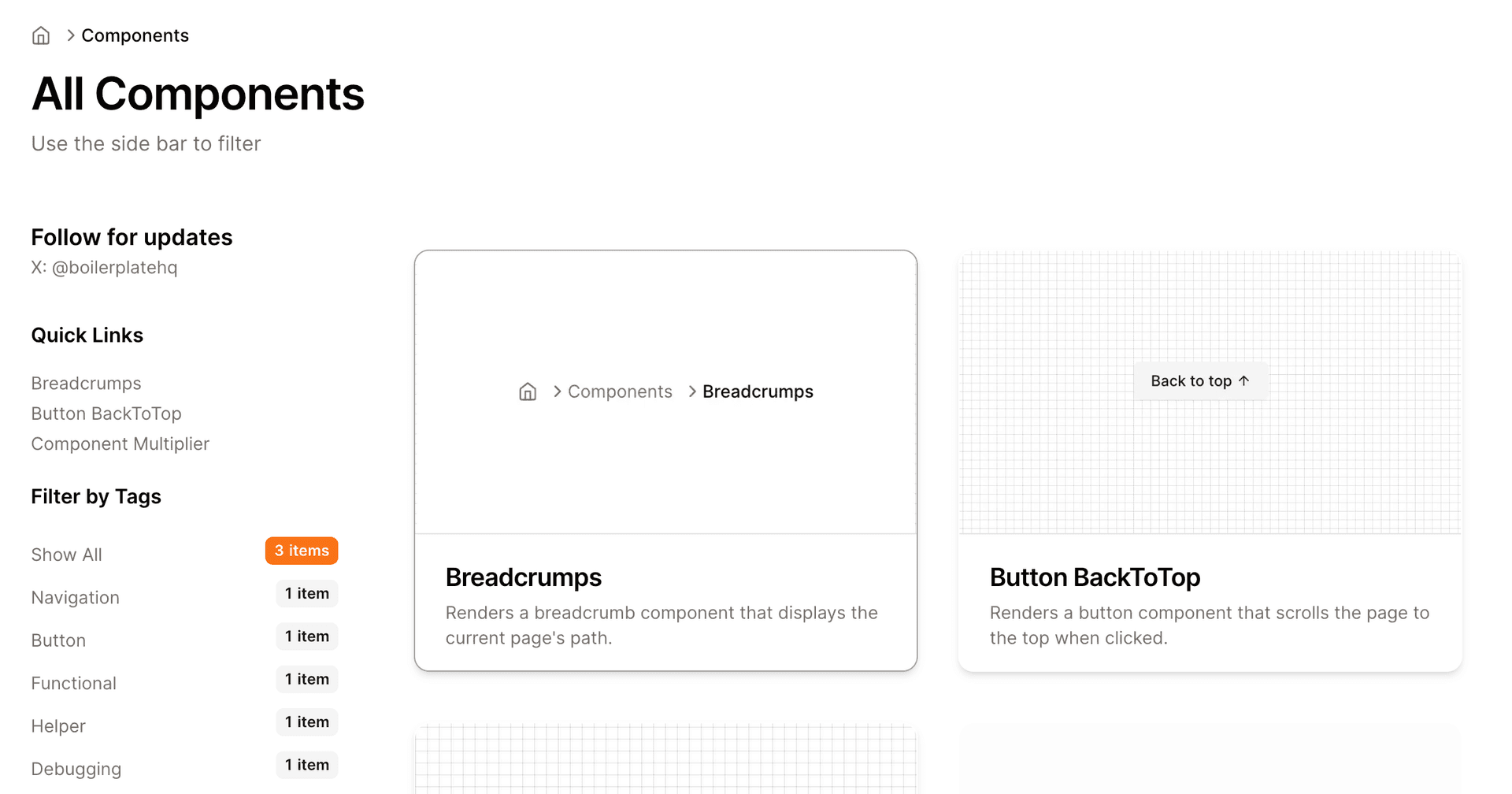
Ui Component Library
Want to create your own Ui Component Library like shadcn/ui? This is your template!
Copy To Clipboard
Copies the given string to the clipboard.
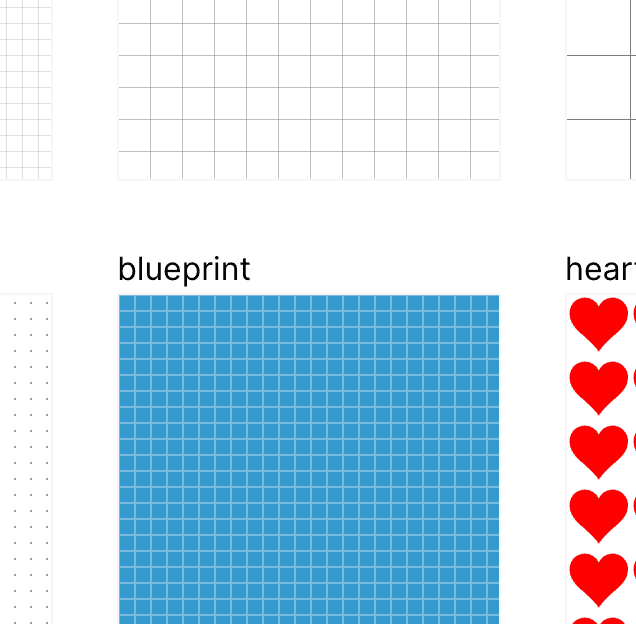
Background Pattern
Provides a background image URL based on a predefined pattern.
Frequently Asked Questions
We have compiled a list of frequently asked questions. If you have any other questions, please do not hesitate to contact us via email or using the chat function. We are here to help!