Copy To Clipboard
Boilerplate for a Typescript function that copies a given string to the clipboard. This is a utility Typescript function that can be copy & pasted into your codebase.
Step-By-Step Guide
1. Copy the Source Code
@/lib/utils.tsx
1/**
2 * Copies the given string to the clipboard.
3 *
4 * @param str - The string to be copied.
5 */
6export function CopyToClipboard(str: string | undefined) {
7 if (!str) return;
8 navigator.clipboard.writeText(str);
9}
@/lib/utils.tsx
1import { toast } from 'sonner';
2
3/**
4 * Copies the given string to the clipboard.
5 *
6 * @param str - The string to be copied.
7 */
8export function CopyToClipboard(str: string | undefined) {
9 if (!str) return;
10 navigator.clipboard
11 .writeText(str)
12 .then(() => {
13 toast.success('String has been copied!');
14 })
15 .catch((err) => {
16 toast.error('Error copying string. Try again please!', err);
17 });
18}
19
20// With Custom Message
21
22/**
23 * Copies the given string to the clipboard.
24 *
25 * @param str - The string to be copied.
26 */
27export function CopyToClipboard(str: string | undefined, message?: string) {
28 if (!str) return;
29 navigator.clipboard
30 .writeText(str)
31 .then(() => {
32 toast.success(message || 'String has been copied!');
33 })
34 .catch((err) => {
35 toast.error('Error copying string. Try again please!', err);
36 });
37}
2. Use in your App
@/components/Button_CopyToClipboard.tsx
1import { CopyToClipboard } from '@/lib/utils';
2import { ClipboardCopy } from 'lucide-react';
3
4export default function Button_CopyToClipboard({
5 text,
6 className,
7}: {
8 text: string | undefined;
9 className?: string;
10}) {
11 return (
12 <button onClick={() => CopyToClipboard(text)}>
13 <span className="sr-only">Copy to Clipboard</span>
14 <ClipboardCopy size={22} />
15 </button>
16 );
17}
Tech Stack
Only the finest ingredients are used in our products. We made sure that we only use the best technologies available which offer a free tier! Yes, you read that right. Everything can be set up for FREE!
You May Also Like
Didn't find what you were looking for? Check out these other items.
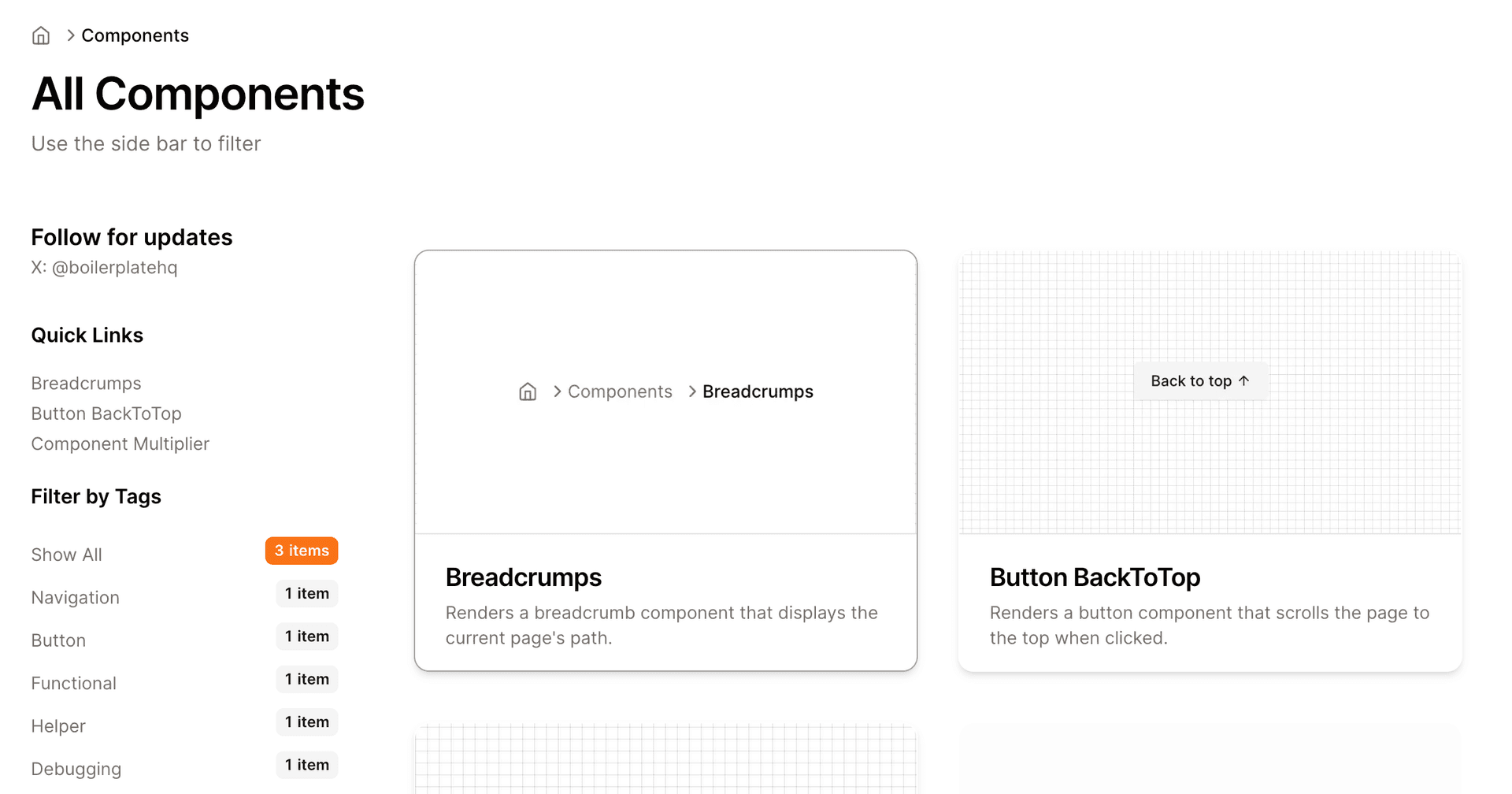
Ui Component Library
Want to create your own Ui Component Library like shadcn/ui? This is your template!
isBase64
Checks if a string is a valid base64 encoded string.
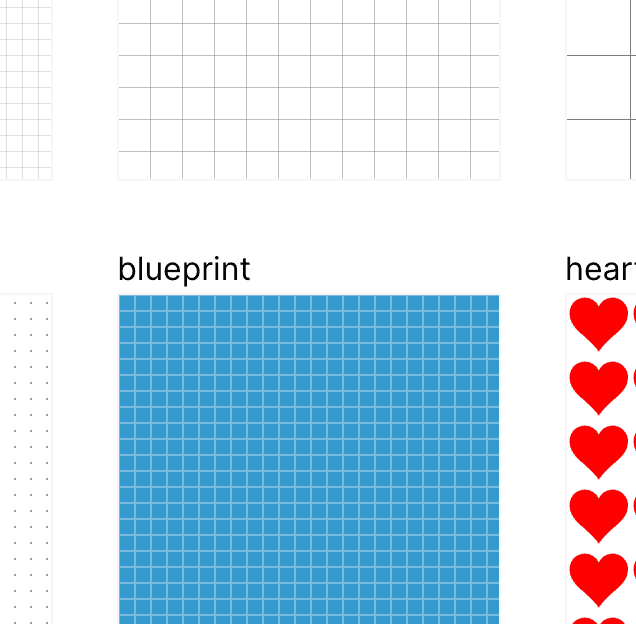
Background Pattern
Provides a background image URL based on a predefined pattern.
Frequently Asked Questions
We have compiled a list of frequently asked questions. If you have any other questions, please do not hesitate to contact us via email or using the chat function. We are here to help!