String To Slug
Turns a string into a slug by spliting the string into an array of words, and joining them with hyphens. This is a utility Typescript function that can be copy & pasted into your codebase.
Step-By-Step Guide
1. Copy the Source Code
@/lib/utils.ts
1/**
2 * Converts a string to a slug.
3 * Replaces non-word characters and underscores with spaces,
4 * splits the string into an array of words, and joins them with hyphens.
5 * Finally, converts the resulting string to lowercase.
6 *
7 * @param string - The string to convert to a slug, such as 'This is a Header!'.
8 * @returns The slugified string, such as this-is-a-header.
9 */
10export function stringToSlug(string: string) {
11 return string
12 .toString()
13 .replace(/[\W_]+/g, ' ')
14 .split(' ')
15 .join('-')
16 .toLowerCase();
17}
2. Use it in a file
/page.tsx
1import { stringToSlug } from '@/lib/utils';
2
3export default function SomeComponent(){
4 const headline = "This function is awesome!"
5 return (
6 <h1 id={stringToSlug(headline)}>{headline}</h1>
7 // Will result in: <h1 id="this-function-is-awesome">This function is awesome!</h1>
8 // So you can use it as a Link Anchor {YOUR_URL}.com/#this-function-is-awesome
9 )
10}
Tech Stack
Only the finest ingredients are used in our products. We made sure that we only use the best technologies available which offer a free tier! Yes, you read that right. Everything can be set up for FREE!
You May Also Like
Didn't find what you were looking for? Check out these other items.
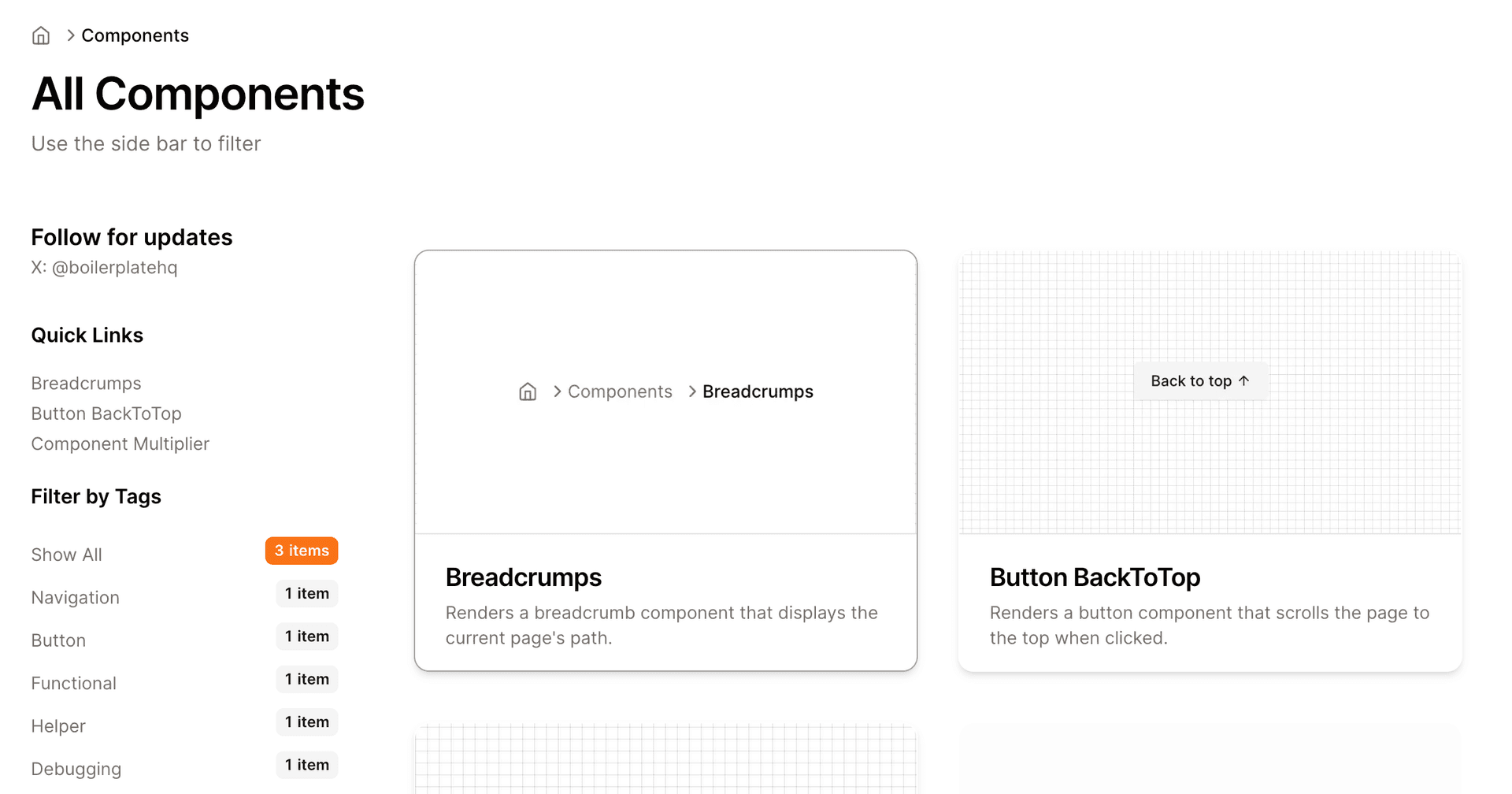
Ui Component Library
Want to create your own Ui Component Library like shadcn/ui? This is your template!
Copy To Clipboard
Copies the given string to the clipboard.
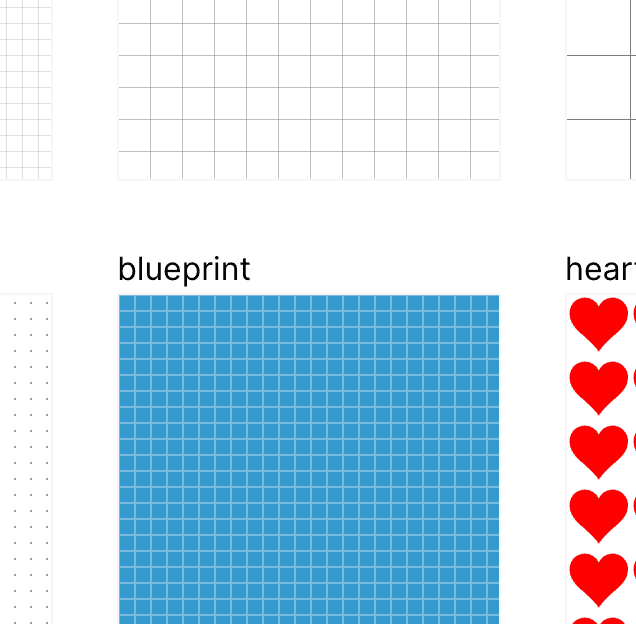
Background Pattern
Provides a background image URL based on a predefined pattern.
Frequently Asked Questions
We have compiled a list of frequently asked questions. If you have any other questions, please do not hesitate to contact us via email or using the chat function. We are here to help!